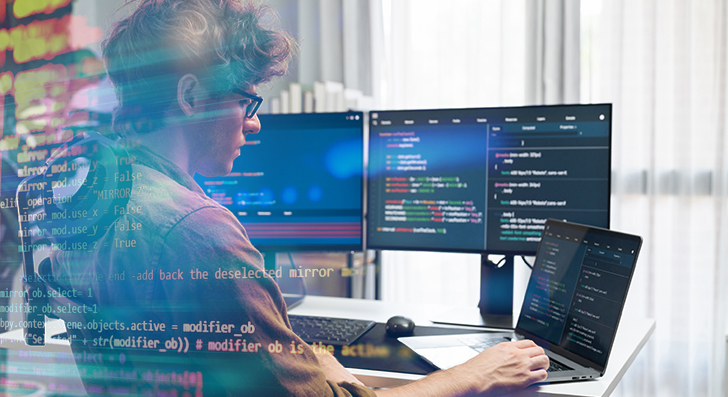
Scalability suggests your software can tackle expansion—far more customers, extra facts, plus much more site visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and anxiety later on. Here’s a transparent and sensible guideline that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later on—it ought to be element within your program from the start. Several purposes fall short every time they improve quick for the reason that the original style and design can’t deal with the additional load. As a developer, you must Imagine early about how your system will behave under pressure.
Get started by creating your architecture being adaptable. Stay away from monolithic codebases wherever every thing is tightly linked. Rather, use modular layout or microservices. These styles break your app into scaled-down, unbiased components. Every single module or company can scale on its own with no influencing the whole program.
Also, think of your databases from working day one. Will it want to manage one million customers or maybe 100? Pick the ideal type—relational or NoSQL—according to how your info will increase. System for sharding, indexing, and backups early, Even when you don’t want them nevertheless.
One more essential stage is in order to avoid hardcoding assumptions. Don’t produce code that only will work less than latest ailments. Give thought to what would happen if your user foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design patterns that assistance scaling, like message queues or occasion-driven methods. These assist your app deal with much more requests with out acquiring overloaded.
Any time you Make with scalability in your mind, you're not just preparing for success—you might be lessening upcoming complications. A properly-prepared technique is easier to maintain, adapt, and mature. It’s superior to get ready early than to rebuild later on.
Use the proper Databases
Picking out the appropriate databases can be a crucial Portion of developing scalable purposes. Not all databases are crafted a similar, and utilizing the Improper one can sluggish you down or perhaps induce failures as your app grows.
Begin by being familiar with your knowledge. Is it really structured, like rows in the table? If Of course, a relational databases like PostgreSQL or MySQL is an effective fit. These are definitely sturdy with relationships, transactions, and regularity. They also assist scaling methods like examine replicas, indexing, and partitioning to deal with much more website traffic and info.
In the event your info is a lot more flexible—like consumer activity logs, merchandise catalogs, or documents—take into account a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at handling substantial volumes of unstructured or semi-structured data and might scale horizontally extra very easily.
Also, look at your study and publish styles. Do you think you're doing a lot of reads with much less writes? Use caching and skim replicas. Are you currently dealing with a significant write load? Explore databases which can handle significant write throughput, and even function-centered data storage methods like Apache Kafka (for short term facts streams).
It’s also good to think ahead. You may not have to have advanced scaling attributes now, but selecting a database that supports them signifies you gained’t will need to modify afterwards.
Use indexing to hurry up queries. Stay clear of unnecessary joins. Normalize or denormalize your knowledge determined by your entry styles. And generally watch databases effectiveness when you improve.
To put it briefly, the ideal databases depends upon your app’s composition, velocity requires, And exactly how you be expecting it to mature. Choose time to select sensibly—it’ll help save many difficulty later.
Optimize Code and Queries
Fast code is key to scalability. As your application grows, each individual compact hold off adds up. Badly created code or unoptimized queries can decelerate overall performance and overload your system. That’s why it’s important to Establish successful logic from the start.
Start by crafting cleanse, straightforward code. Steer clear of repeating logic and take away nearly anything unneeded. Don’t select the most complicated Alternative if an easy a single works. Maintain your functions shorter, centered, and easy to check. Use profiling resources to uncover bottlenecks—sites the place your code requires much too prolonged to run or works by using a lot of memory.
Future, have a look at your databases queries. These typically slow factors down over the code alone. Ensure Each individual query only asks for the info you actually will need. Steer clear of Pick out *, which fetches every thing, and as a substitute select distinct fields. Use indexes to speed up lookups. And prevent performing too many joins, Primarily across massive tables.
If you recognize the exact same information staying asked for repeatedly, use caching. Keep the results briefly working with tools like Redis or Memcached which means you don’t really need to repeat highly-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in groups. This cuts down on overhead and tends to make your application more effective.
Remember to examination with substantial datasets. Code and queries that do the job fine with 100 information may well crash whenever they have to take care of one million.
To put it briefly, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These methods enable your software continue to be clean and responsive, at the same time as the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's got to handle more users and much more visitors. If every thing goes by means of a single server, it's going to swiftly become a bottleneck. That’s exactly where load balancing and caching come in. Both of these applications enable keep the application rapid, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Instead of one server accomplishing the many get the job done, the load balancer routes end users to distinct servers according to availability. This means no single server receives overloaded. If just one server goes down, the load balancer can ship traffic to the Many others. Instruments like Nginx, HAProxy, or cloud-based mostly options from AWS and Google Cloud make this easy to build.
Caching is about storing knowledge temporarily so it might be reused speedily. When customers ask for a similar data once more—like an item webpage or a profile—you don’t should fetch it from your databases whenever. You are able to provide it from your cache.
There's two widespread types of caching:
one. Server-aspect caching (like Redis or Memcached) stores knowledge in memory for fast entry.
2. Customer-facet caching (like browser caching or CDN caching) merchants static data files near to the person.
Caching decreases databases load, improves pace, and makes your application much more successful.
Use caching for things that don’t modify normally. And often be certain your cache is up to date when facts does change.
In a nutshell, load balancing and caching are very simple but effective instruments. Together, they help your application manage additional users, remain rapid, and recover from difficulties. If you intend to mature, you'll need equally.
Use Cloud and Container Applications
To construct scalable programs, you require applications that let your app expand conveniently. That’s exactly where cloud platforms and containers are available in. They provide you overall flexibility, cut down setup time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Permit you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess upcoming potential. When traffic increases, you are able to include a lot more assets with only a few clicks or instantly employing automobile-scaling. When targeted traffic drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You'll be able to center on constructing your app as opposed to handling infrastructure.
Containers are An additional key tool. A container offers your application and every little thing it must run—code, libraries, configurations—into one particular unit. This makes it quick to maneuver your app among environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Whenever your app uses many containers, equipment like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part of your respective app crashes, it restarts it automatically.
Containers also help it become simple to separate aspects of your app into services. You may update or scale components independently, which happens to be great for overall performance and trustworthiness.
In brief, applying cloud and container equipment usually means it is possible to scale fast, deploy simply, and recover speedily when problems come about. If you would like your application to expand without the need of limitations, start out utilizing these instruments early. They save time, minimize hazard, and assist you to keep centered on developing, not repairing.
Observe Every little thing
When you don’t monitor your application, you gained’t know when points go wrong. Checking allows you see how your app is doing, location issues early, and make far better selections as your application grows. It’s a vital A part of creating scalable devices.
Get started by tracking fundamental metrics like CPU usage, memory, disk Room, and reaction time. These inform you how your servers and products and services are performing. Resources like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—check your app way too. Control just how long it will require for people to load internet pages, how frequently glitches materialize, and where by they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Build alerts for significant complications. Such as, In the event your response time goes above a Restrict or maybe a check here assistance goes down, it is best to get notified promptly. This will help you correct concerns quickly, frequently before users even see.
Checking is additionally helpful whenever you make changes. For those who deploy a different attribute and see a spike in faults or slowdowns, you may roll it back again before it leads to real problems.
As your app grows, traffic and facts boost. With out checking, you’ll overlook signs of difficulties until finally it’s too late. But with the appropriate resources set up, you remain on top of things.
In a nutshell, checking will help you keep your application reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it really works well, even under pressure.
Remaining Ideas
Scalability isn’t only for huge providers. Even tiny applications require a robust Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you can Construct applications that develop efficiently without the need of breaking under pressure. Start off small, Feel major, and Develop sensible.